📜 [專欄新文章] Reason Why You Should Use EIP1167 Proxy Contract. (With Tutorial)
✍️ Ping Chen
📥 歡迎投稿: https://medium.com/taipei-ethereum-meetup #徵技術分享文 #使用心得 #教學文 #medium
EIP1167 minimal proxy contract is a standardized, gas-efficient way to deploy a bunch of contract clones from a factory.
1. Who may consider using EIP1167
For some DApp that are creating clones of a contract for its users, a “factory pattern” is usually introduced. Users simply interact with the factory to get a copy. For example, Gnosis Multisig Wallet has a factory. So, instead of copy-and-paste the source code to Remix, compile, key in some parameters, and deploy it by yourself, you can just ask the factory to create a wallet for you since the contract code has already been on-chain.
The problem is: we need standalone contract instances for each user, but then we’ll have many copies of the same bytecode on the blockchain, which seems redundant. Take multisig wallet as an example, different multisig wallet instances have separate addresses to receive assets and store the wallet’s owners’ addresses, but they can share the same program logic by referring to the same library. We call them ‘proxy contracts’.
One of the most famous proxy contract users is Uniswap. It also has a factory pattern to create exchanges for each ERC20 tokens. Different from Gnosis Multisig, Uniswap only has one exchange instance that contains full bytecode as the program logic, and the remainders are all proxies. So, when you go to Etherscan to check out the code, you’ll see a short bytecode, which is unlikely an implementation of an exchange.
0x3660006000376110006000366000732157a7894439191e520825fe9399ab8655e0f7085af41558576110006000f3
What it does is blindly relay every incoming transaction to the reference contract 0x2157a7894439191e520825fe9399ab8655e0f708by delegatecall.
Every proxy is a 100% replica of that contract but serving for different tokens.
The length of the creation code of Uniswap exchange implementation is 12468 bytes. A proxy contract, however, has only 46 bytes, which is much more gas efficient. So, if your DApp is in a scenario of creating copies of a contract, no matter for each user, each token, or what else, you may consider using proxy contracts to save gas.
2. Why use EIP1167
According to the proposal, EIP is a “minimal proxy contract”. It is currently the known shortest(in bytecode) and lowest gas consumption overhead implementation of proxy contract. Though most ERCs are protocols or interfaces, EIP1167 is the “best practice” of a proxy contract. It uses some EVM black magic to optimize performance.
EIP1167 not only minimizes length, but it is also literally a “minimal” proxy that does nothing but proxying. It minimizes trust. Unlike other upgradable proxy contracts that rely on the honesty of their administrator (who can change the implementation), address in EIP1167 is hardcoded in bytecode and remain unchangeable.
That brings convenience to the community.
Etherscan automatically displays code for EIP1167 proxies.
When you see an EIP1167 proxy, you can definitely regard it as the contract that it points to. For instance, if Etherscan finds a contract meets the format of EIP1167, and the reference implementation’s code has been published, it will automatically use that code for the proxy contract. Unfortunately, non-standard EIP1167 proxies like Uniswap will not benefit from this kind of network effect.
3. How to upgrade a contract to EIP1167 compatible
*Please read all the steps before use, otherwise there might have problems.
A. Build a clone factory
For Vyper, there’s a function create_with_code_of(address)that creates a proxy and returns its address. For Solidity, you may find a reference implementation here.
function createClone(address target) internal returns (address result){ bytes20 targetBytes = bytes20(target); assembly { let clone := mload(0x40) mstore(clone, 0x3d602d80600a3d3981f3363d3d373d3d3d363d73000000000000000000000000) mstore(add(clone, 0x14), targetBytes) mstore(add(clone, 0x28), 0x5af43d82803e903d91602b57fd5bf30000000000000000000000000000000000) result := create(0, clone, 0x37) }}
You can either deploy the implementation contract first or deploy it with the factory’s constructor. I’ll suggest the former, so you can optimize it with higher runs.
contract WalletFactory is CloneFactory { address Template = "0xc0ffee"; function createWallet() external returns (address newWallet) { newWallet = createClone(Template); }}
B. Replace constructor with initializer
When it comes to a contract, there are two kinds of code: creation code and runtime code. Runtime code is the actual business logic stored in the contract’s code slot. Creation code, on the other hand, is runtime code plus an initialization process. When you compile a solidity source code, the output bytecode you get is creation code. And the permanent bytecode you can find on the blockchain is runtime code.
For EIP1167 proxies, we say it ‘clones’ a contract. It actually clones a contract’s runtime code. But if the contract that it is cloning has a constructor, the clone is not 100% precise. So, we need to slightly modify our implementation contract. Replace the constructor with an ‘initializer’, which is part of the permanent code but can only be called once.
// constructorconstructor(address _owner) external { owner = _owner;}// initializerfunction set(address _owner) external { require(owner == address(0)); owner = _owner;}
Mind that initializer is not a constructor, so theoretically it can be called multiple times. You need to maintain the edge case by yourself. Take the code above as an example, when the contract is initialized, the owner must never be set to 0, or anyone can modify it.
C. Don’t assign value outside a function
As mentioned, a creation code contains runtime code and initialization process. A so-called “initialization process” is not only a constructor but also all the variable assignments outside a function. If an EIP1167 proxy points to a contract that assigns value outside a function, it will again have different behavior. We need to remove them.
There are two approaches to solve this problem. The first one is to turn all the variables that need to be assigned to constant. By doing so, they are no longer a variable written in the contract’s storage, but a constant value that hardcoded everywhere it is used.
bytes32 public constant symbol = "4441490000000000000000000000000000000000000000000000000000000000";uint256 public constant decimals = 18;
Second, if you really want to assign a non-constant variable while initializing, then just add it to the initializer.
mapping(address => bool) public isOwner;uint public dailyWithdrawLimit;uint public signaturesRequired;
function set(address[] _owner, uint limit, uint required) external { require(dailyWithdrawLimit == 0 && signaturesRequired == 0); dailyWithdrawLimit = limit; signaturesRequired = required; //DO SOMETHING ELSE}
Our ultimate goal is to eliminate the difference between runtime code and creation code, so EIP1167 proxy can 100% imitate its implementation.
D. Put them all together
A proxy contract pattern splits the deployment process into two. But the factory can combine two steps into one, so users won’t feel different.
contract multisigWallet { //wallet interfaces function set(address[] owners, uint required, uint limit) external;}contract walletFactory is cloneFactory { address constant template = "0xdeadbeef"; function create(address[] owners, uint required, uint limit) external returns (address) { address wallet = createClone(template); multisigWallet(wallet).set(owners, required, limit); return wallet; }}
Since both the factory and the clone/proxy has exactly the same interface, no modification is required for all the existing DApp, webpage, and tools, just enjoy the benefit of proxy contracts!
4. Drawbacks
Though proxy contract can lower the storage fee of deploying multiple clones, it will slightly increase the gas cost of each operation in the future due to the usage of delegatecall. So, if the contract is not so long(in bytes), and you expect it’ll be called millions of times, it’ll eventually be more efficient to not use EIP1167 proxies.
In addition, proxy pattern also introduces a different attack vector to the system. For EIP1167 proxies, trust is minimized since the address they point to is hardcoded in bytecode. But, if the reference contract is not permanent, some problems may happen.
You might ever hear of parity multisig wallet hack. There are multiple proxies(not EIP1167) that refer to the same implementation. However, the wallet has a self-destruct function, which empties both the storage and the code of a contract. Unfortunately, there was a bug in Parity wallet’s access control and someone accidentally gained the ownership of the original implementation. That did not directly steal assets from other parity wallets, but then the hacker deleted the original implementation, making all the remaining wallets a shell without functionality, and lock assets in it forever.
https://cointelegraph.com/news/parity-multisig-wallet-hacked-or-how-come
Conclusion
In brief, the proxy factory pattern helps you to deploy a bunch of contract clones with a considerably lower gas cost. EIP1167 defines a bytecode format standard for minimal proxy and it is supported by Etherscan.
To upgrade a contract to EIP1167 compatible, you have to remove both constructor and variable assignment outside a function. So that runtime code will contain all business logic that proxies may need.
Here’s a use case of EIP1167 proxy contract: create adapters for ERC1155 tokens to support ERC20 interface.
pelith/erc-1155-adapter
References
https://eips.ethereum.org/EIPS/eip-1167
https://blog.openzeppelin.com/on-the-parity-wallet-multisig-hack-405a8c12e8f7/
Donation:
pingchen.eth
0xc1F9BB72216E5ecDc97e248F65E14df1fE46600a
Reason Why You Should Use EIP1167 Proxy Contract. (With Tutorial) was originally published in Taipei Ethereum Meetup on Medium, where people are continuing the conversation by highlighting and responding to this story.
👏 歡迎轉載分享鼓掌
同時也有7部Youtube影片,追蹤數超過23萬的網紅Hey It's Dena,也在其Youtube影片中提到,Introducing Aden! OPEN FOR MORE INFO 打開我有更多資訊 __ ✧ Extra Notes 補充內容 第一次聽到ADEN的歌『想了你6次』實在是太愛了每聽就會粉紅泡泡那種!於是問他我可不可以cover~沒想到他直接讓我寫全新女生版的主歌!!!希望你們喜歡啦~ ...
「go build 教學」的推薦目錄:
- 關於go build 教學 在 Taipei Ethereum Meetup Facebook 的最佳貼文
- 關於go build 教學 在 Taipei Ethereum Meetup Facebook 的最佳解答
- 關於go build 教學 在 筆尖溫度 Facebook 的精選貼文
- 關於go build 教學 在 Hey It's Dena Youtube 的精選貼文
- 關於go build 教學 在 FriesBro Youtube 的精選貼文
- 關於go build 教學 在 Wooden Ren Youtube 的最讚貼文
- 關於go build 教學 在 Go Build | Carson Blog 的評價
- 關於go build 教學 在 用GitHub Actions 部署Go 語言服務 - 小惡魔 的評價
- 關於go build 教學 在 Golang 簡介、安裝、與快速開始By 彭彭 - YouTube 的評價
- 關於go build 教學 在 golang教學的推薦與評價,FACEBOOK和網紅們這樣回答 的評價
go build 教學 在 Taipei Ethereum Meetup Facebook 的最佳解答
📜 [專欄新文章] 參加 Crosslink 2019 Taiwan 一探究竟那些不可錯過的以太坊最新發展 (第二天議程)
✍️ Phini Yang
📥 歡迎投稿: https://medium.com/taipei-ethereum-meetup #徵技術分享文 #使用心得 #教學文 #medium
解析以太坊上的安全狀態通道 (A Secure State Channels Framework for Ethereum)— Liam Horne, Co-founder of L4 Ventures & ETHGlobal
#Intermediate
擴展性 Scalability 一直是近年以太坊生態系中重點任務。第二層協定 Layer 2採取的解決方向是用已有容量來處理更多交易,並不是增加以太坊本身容量,而是應用程式流量優化與用戶操作流程簡化等方式達到目的。它不會對基層協議做出任何更改,僅使用智能合約與鏈下應用程式交互。
「狀態通道 State Channel」則是第二層協議的其中之一解法,是一種透過鏈下交易與更新狀態的技術,在重複支付或遊戲加速兩項表現優異。在 L4 Ventures 中,開發人員可透過通用模塊化來快速建構狀態通道。此演講將帶你了解 State Channel 未來整體佈局。
延伸閱讀:Counterfactual: Generalized State Channels on Ethereum By Liam Horne Layer 2: From Payment to Generalized State Channel By 陳品
如何打造一般化的 Layer2 應用服務 (How to Build Generalized Application on Layers2)— Yuriko Nishijima, Developer & Researcher of Cryptoeconomics Lab
#Intermediate
「電漿網路 Plasma」是第二層協議的另一個解法,它創建附加在以太坊主鏈上的子鏈,並運用此技術實現狀態通道效果,特別在支付應用上見長。
Plasma 是 2017 年 Vitalik 跟 Joseph Poon (閃電網路提案人之一) 提出的方案,並於 2018 年有爆炸性成長。Cryptoeconomics Lab 在 Plasma 深耕已久,也正在實作這項協定,透過演講你會瞭解 Plasma 擴容方案將如何實現。
掌控你的私鑰與隱私 (Own Your Private Keys and Privacy) — Hankuan Yu, Head of Engineering & Hank Chiu of Engineering of HTC EXODUS
#Beginner
在區塊鏈世界中,私鑰就是一切。HTC Exodus 是全世界第一款使用硬體保護私鑰的區塊鏈手機,從使用者最根本問題出發。今年 9月 HTC 更提出新一代私鑰還原架構 — SKR 2.0,進一步提升私鑰還原之安全性。接下來,在私鑰安全及區塊鏈隱私上,HTC 將有什麼進一步規劃呢?
模組化的點對點網路協議 (libp2p: Modular Peer-to-Peer Networking Stack) — Raùl Kripalani, libp2p Tech Lead of Protocol Labs
#Intermediate #Eth2.0 #Go Language
在區塊鏈專案的網路層協議之中,近年來最值得關注的為 libp2p project,它是 IPFS 網路協議之延伸,現已另成一獨立網路協議專案,並由 Protocol Labs 團隊來維護。在去中心化場景中,為了解決節點與節點之間訪問的各種問題,libp2p 提供了解決方案,並將節點能在多個網絡中共享,大家亦能受惠於此。況且 libp2p 更提供可讓開發者快速使用的模組化通用包,廣受區塊鏈開發團隊青睞。
延伸閱讀:Why libp2p? By Pierre KriegerUnderstanding IPFS in Depth(5/6): What is Libp2p? By Vasa
剖析以太坊 2.0 客戶端 (The anatomy of a basic Ethereum 2.0 client) — Adrian Manning, Co-founder of Sigma Prime
#Intermediate #Eth2.0 #Rust Language
Lighthouse 是 Sigma Prime 針對 Eth2.0 客戶端所開發的專案,選用新一代語言 Rust 來做開發。Sigma Prime 於早期就參與 Casper 研究跟實作,對 Eth 2.0 核心的共識機制相當熟捻,而共同創辦人 Adrian 更是網路安全跟密碼學的專家。
講者介紹:Adrian Manning, Co-Founder of Sigma Prime▪ PhD in Quantum Field Theory▪ Cyber/Cryptography expert
延伸閱讀:Casper FFG:以實現權益證明為目標的共識協定 By Juin
次世代的以太坊虛擬機 (eWASM VM — The next generation Ethereum Virtual Machine )— Hung-Ying Tai, VP of engineering of Second State
#Intermediate #Eth2.0 #Virtual Machine
以太坊為了迎接下一代的虛擬機 — eWASM VM (Ethereum Flavored WebAssembly Virtual Machine),正如火如荼地開發 Solidity 的 eWASM 後端銜接。「eWASM 虛擬機」顧名思義將不再採用現在黃皮書中的指令集 ,而是使用 EWASM 來取代。EWASM 將會強化以太坊虛擬機的效能與安全性,也可以相容更多工具鏈,可以做到用一般程式語言如 C / C++、Go、Rust 來寫合約。
延伸閱讀:https://github.com/ewasm/design/blob/master/rationale.md
Geth 上的新型 BFT 共識演算法 (New A New 2-Step BFT Consensus Algorithm in geth)— Tung-Wei Kuo, Assistant Professor of National Chengchi University
#Advanced #Academic
傳統的拜占庭容錯 BFT (Byzantine Fault Tolerance) 共識演算法需要三個步驟來完成共識。在這場演講中,Tung Wei 將提出一個兩步驟 TwoStepBFT 的優化算法,在容許錯誤節點下,亦同時保有安全及活性。
使用以太坊 Proof-of-Authority 聯盟鏈進行跨醫院的資料共享 (Ethereum PoA Consortium Chain to Support Inter-Hospital Data Sharing) — Kung Chen, Professor of National Chengchi University
#Intermediate #Academic
目前社會中尚缺乏一套完善的整合系統,可供民眾進行醫療資訊的授權與共享。若病歷能有效地共享與授權,將可強化醫療分級轉診服務等下一代醫療服務。偏偏這些醫療資訊牽涉民眾隱私權等敏感議題,國立政治大學陳恭教授將分享如何透過區塊鏈對資料授權能力,來提升使用者的資料自主權,達成有效進行交換醫療資訊的目標。
更多資訊請直接參考官網議程:https://crosslink.taipei/schedule/2019-10–20
參加 Crosslink 2019 Taiwan 一探究竟那些不可錯過的以太坊最新發展 (第二天議程) was originally published in Taipei Ethereum Meetup on Medium, where people are continuing the conversation by highlighting and responding to this story.
👏 歡迎轉載分享鼓掌
go build 教學 在 筆尖溫度 Facebook 的精選貼文
【筆尖碎碎念】😜
有經營粉絲團的朋友,一定都有感覺,
2017年中過後,觸及率都有一定程度的下降....😓
原來FB的演算法在CEO馬克的宣示下,
未來將以朋友、家人👫的社交貼文為主,
意思是粉絲團貼文在動態牆上的比例將會降低。
作為一個使用者,我覺得這是好的方向,
要不然我個人的動態牆都快被一些媒體新聞淹沒了,
但作為一個粉絲團的版主可就苦惱了,
這樣的改變意味著作品的機率降低了....📉
因應這樣的趨勢與改變,
如果你想要多看 筆尖溫度 的教學與作品貼文,
有兩個方法你可以做👇
1. 點選粉絲頁上方" 追蹤中 "的按紐,設定為"搶先看"!然後如果看到貼文的時多互動一下,按個讚、留言或分享。
2. 關注訂閱 筆尖溫度 其他社群的頻道:
Instagram ( 照片與短片為主 )
👉 https://www.instagram.com/nib01tw/
痞客邦 ( 有比較詳細的圖片步驟解說 )
👉 https://goo.gl/mLa3qf
微博 ( 以簡體字發表 )
👉 https://goo.gl/VwBZ8k
Youtube頻道 ( 可收看高畫質影音 )
👉 https://goo.gl/HEu2JB
筆尖溫度社團 ( 討論分享大家的作品 )
👉 https://goo.gl/67NczW
#碎碎念 #筆尖溫度
One of our big focus areas for 2018 is making sure the time we all spend on Facebook is time well spent.
We built Facebook to help people stay connected and bring us closer together with the people that matter to us. That's why we've always put friends and family at the core of the experience. Research shows that strengthening our relationships improves our well-being and happiness.
But recently we've gotten feedback from our community that public content -- posts from businesses, brands and media -- is crowding out the personal moments that lead us to connect more with each other.
It's easy to understand how we got here. Video and other public content have exploded on Facebook in the past couple of years. Since there's more public content than posts from your friends and family, the balance of what's in News Feed has shifted away from the most important thing Facebook can do -- help us connect with each other.
We feel a responsibility to make sure our services aren’t just fun to use, but also good for people's well-being. So we've studied this trend carefully by looking at the academic research and doing our own research with leading experts at universities.
The research shows that when we use social media to connect with people we care about, it can be good for our well-being. We can feel more connected and less lonely, and that correlates with long term measures of happiness and health. On the other hand, passively reading articles or watching videos -- even if they're entertaining or informative -- may not be as good.
Based on this, we're making a major change to how we build Facebook. I'm changing the goal I give our product teams from focusing on helping you find relevant content to helping you have more meaningful social interactions.
We started making changes in this direction last year, but it will take months for this new focus to make its way through all our products. The first changes you'll see will be in News Feed, where you can expect to see more from your friends, family and groups.
As we roll this out, you'll see less public content like posts from businesses, brands, and media. And the public content you see more will be held to the same standard -- it should encourage meaningful interactions between people.
For example, there are many tight-knit communities around TV shows and sports teams. We've seen people interact way more around live videos than regular ones. Some news helps start conversations on important issues. But too often today, watching video, reading news or getting a page update is just a passive experience.
Now, I want to be clear: by making these changes, I expect the time people spend on Facebook and some measures of engagement will go down. But I also expect the time you do spend on Facebook will be more valuable. And if we do the right thing, I believe that will be good for our community and our business over the long term too.
At its best, Facebook has always been about personal connections. By focusing on bringing people closer together -- whether it's with family and friends, or around important moments in the world -- we can help make sure that Facebook is time well spent.
go build 教學 在 Hey It's Dena Youtube 的精選貼文
Introducing Aden!
OPEN FOR MORE INFO 打開我有更多資訊
__
✧ Extra Notes 補充內容
第一次聽到ADEN的歌『想了你6次』實在是太愛了每聽就會粉紅泡泡那種!於是問他我可不可以cover~沒想到他直接讓我寫全新女生版的主歌!!!希望你們喜歡啦~
想了你6次 Dena 版 Version
music & lyrics by Aden & Dena
Baby come here to be my only
我受夠了只是想妳 這道選擇題他很簡單
Money 再多我也選擇妳 我|在這里等妳
喔! 請妳不要想太多 簡單點請妳跟我說
Is it real or tell me if it’s all just in my head
Oh I can’t sleep, cus every time I start to think about us
The way you grabbed my hand and told me
You wanna go out and let’s eat
I can’t breathe cus you’re exactly what I need aye
They say I’m falling too fast
But I don’t mind
Cus it you that I’m falling into you
Something about you makes me feel
Like we can build sth brand new
Maybe
I’m exactly what you need aye
Baby come here to be my only
我受夠了只是想妳 這道選擇題他很簡單
Money 再多我也選擇妳 我|在這里等妳
喔! 請妳不要想太多 簡單點請妳跟我說
喔 那麼多的feelings get involved
喔 我纏了線讓妳操控我
喔 今天想了妳6次
哎 我可不想裝沒事
So 請妳不要想太多 我在這, 等妳跟我說
Music Prod: ADEN
Video Prod: Dena
__
✧ What's On Me 我身上的
Suit Set 套裝:
https://www.pazzo.com.tw/zh-tw/market/n/18694?c=41853
__
✧ Listen to my original“July” 來聽Dena的新歌
Youtube MV: https://youtu.be/sEJypi8BIls
Spotify: https://tinyurl.com/u83d2ckk
KKBOX: https://tinyurl.com/3sr2bd76
iTunes: https://tinyurl.com/zjuvsc7d
Apple Music: https://tinyurl.com/zjuvsc7d
__
✧ Social Medias 社群
INSTAGRAM
https://www.instagram.com/dena_chang/ @dena_chang
FACEBOOK 粉專
https://www.facebook.com/denachmusic
__
✧ Business Inquiries 工作洽談
[email protected]
__
✧ About Dena 有關Dena
哈囉哈囉~叫我Dena就好~我平常喜歡唱歌寫歌(歡迎上網找我的作品們!也有寫給別的歌手唱喔)拍拍影片上傳到Youtube!喜歡可以讓自己變美或是讓心情變美的一切事物!也喜歡一個人在房間跳舞。雖然沒有很厲害但是感覺認真練起來會不得了!歡迎來到我的世界!耶!很開心認識大家!
Hey peeps! It's Dena here! I'm a singer/songwriter/producer based in Taiwan! Ok and apparently I also make videos about what I love and my life! Welcome to my world!!
#想了你6次 #cover
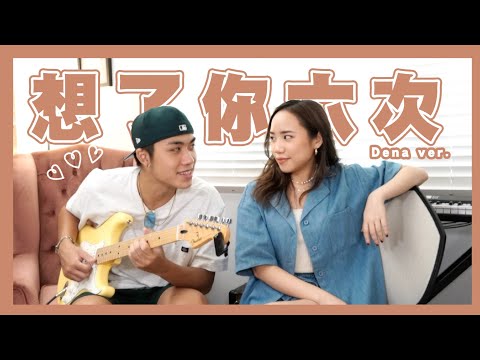
go build 教學 在 FriesBro Youtube 的精選貼文
哈囉 我是大薯
今天要介紹一位
從英雄聯盟S4白金到S10大師 S11鑽1的玩家
他本來就喜歡玩版本強勢角
在悟空小重製改版試玩後就被吸引住開始專精
他S10單雙上路悟空414場59%勝率
▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬
👉幫助達到70,000 訂閱▶ http://bit.ly/2ItnvA6
👉公開Line群: https://reurl.cc/Xkzyja
👉LBRY: https://lbry.tv/@FriesBro:2
👉Discord: https://discord.gg/X8Pft8X
👉Facebook粉絲團: https://www.facebook.com/heroclub2.0/
▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬
👉時間軸
0:00 - 玩家介紹 Player Introduce
0:19 - 玩家配置 Build
0:49 - 觀念技巧Tips
1:31 - 團隊意識 TeamFight
2:03 - 精彩操作 MONTAGE
👉BGM
Track: Mariline - Old Days (ft. Robbie Hutton)
Music Provided by Magic Records
Listen To The Original: https://youtu.be/b9reSIQJSMM
Free Download: https://fanlink.to/eUPu
Usage Policy: http://www.magicmusicLLC.com
Track: Jay Bird - Let Me Go (feat Shiah Maisel)
Music Provided by Magic Records
Listen To The Original: https://youtu.be/V82xl1xLDWM
Free Download: https://fanlink.to/eTh8
Usage Policy: http://www.magicmusicLLC.com
Track: Coopex - Memories (ft. Amanda Yang)
Music Provided by Magic Records
Listen To The Original: https://youtu.be/ccB-UoyTG80
Free Download: https://fanlink.to/dhU3
▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬
歡迎投稿個人精彩操作👉https://m.me/heroclub2.0
LOL錄製&投稿教學👉https://youtu.be/aKkDWMG3vGk
投稿短片請加入Line群👉 https://reurl.cc/Xkzyja
▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬
#大薯

go build 教學 在 Wooden Ren Youtube 的最讚貼文
加入木工線上課程等候名單:https://woodenren.com/b2f7
HI,同學們好,大家可能會有疑惑,網路上的木工視頻這麼多,為什麼還需要花錢購買線上木工課程呢?
第一點
木工是很專業的一門技術,要做好榫卯家具,有非常多的技巧與細節要注重,如果要在Youtube這樣的平台拍攝很詳細的教學影片,那麼影片的時數將會非常的壟長,長時間的影片就會影響觀看者的意願,會間接導致影片的推播率下降,導致拍攝者花了大量時間心力拍攝的影片無法有效地轉化成實質回饋,講白點就是廣告費極低,所以沒什麼人會願意這麼做的。因此多數的木工影片都是草草帶過有看沒有懂。
第二點
免費的線上影片沒有完整的課程規劃、內容零散,讓有心學習木工的同學,難以全面的學習,間接浪費了寶貴時間與多走不少冤枉路。
第三點
木匠養成學院在100%完成後,仍會繼續更新新課程,通過學員的回饋新增課程內容,當然在屆時課程費用勢必會再調漲,但已經購買的同學是可免費觀看,不會在額外收費,這也是為了感謝同學的支持。
第四點
我遇過不少同學,曾上過各個木工房的木工課程後,過不久就將學習過的木工知識與技巧忘的一乾二淨。如果是線上課程就可重複觀看吸收,不會有學習時限的問題。
加入木工線上課程等候名單 可接收課程製作最新進度:https://woodenren.com/b2f7
▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬
👉Follow me
▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬▬
木工榫接基礎班:https://woodenren.com/course
木頭仁官網 :https://woodenren.com/
Instargram :https://www.instagram.com/wooden_ren/
pinkoi設計商品 :https://www.pinkoi.com/store/moodywoodydesign
木頭仁社團 :https://www.facebook.com/groups/WoodenRen/

go build 教學 在 用GitHub Actions 部署Go 語言服務 - 小惡魔 的推薦與評價
測試: Unit Testing 多一層保護編譯: 透過go build 編譯出Binary 檔案上傳: 寫Dockerfile 將Binary 包進容器內啟動: 透過docker-compose 方式來更新 ... ... <看更多>
go build 教學 在 Go Build | Carson Blog 的推薦與評價
ldflags 我建議如果要打包執行檔出去,用這個指令go build -ldflags "-H=windowsgui -s -w" 不顯示console視窗的方法利用選項: -ldflags ... ... <看更多>